- Published on
How is this blog set up
- Website Introduction
- MDX Introduction and Usage
- Website Color Scheme Settings
- Table of Contents Component
- Blog Layout
- Traffic Analysis
- Comment System
- Code Highlighting
- References system
- LaTeX Render
Website Introduction
This website is built using Next.js + Tailwind CSS + MDX, forked fromtailwind-nextjs-starter-blog
MDX Introduction and Usage
What is MDX?
MDX is a syntax that combines Markdown with JSX, allowing the use of JSX components within Markdown. This enables the utilization of React components in Markdown, providing greater flexibility in content presentation.
The idea of embedding JSX into Markdown is indeed intriguing, as it allows us to use React components within Markdown. This means we can incorporate interactive components into our Markdown content, leading to a more dynamic and flexible presentation of information.
For example, in the aforementioned code, <QuickSortAnimation />
is a React component that can be used directly in Markdown. It presents the process of quick sorting through an interactive animation.
Similarly, MDX allows you to write HTML directly, as shown below:
<video controls>
<source
src="https://github-production-user-asset-6210df.s3.amazonaws.com/28362229/258559849-2124c81f-b99d-4431-839c-347e01a2616c.webm"
type="video/webm"
/>
</video>
Website Color Scheme Settings
The website's color scheme settings are located in the tailwind.config.js
file, which you can modify according to your own preferences.
theme: {
colors: {
primary: colors.sky,
gray: colors.neutral,
...
}
...
}
Table of Contents Component
The toc variable, containing all top-level headings from the document, is passed into the MDX file and can be styled accordingly. To simplify the process of generating a Table of Contents (TOC), you can utilize the existing TOCInline component.
Blog Layout
Setting the Template
Mdx blog content can be mapped to layout components through configuring the frontmatter fields. The layout field will be used to specify which layout component should be used, and all layout templates are found in the ./layouts directory.
A simplest custom layout would be:
export default function ExampleLayout({ frontMatter, children }) {
const { date, title } = frontMatter
return (
<SectionContainer>
<div>{date}</div>
<h1>{title}</h1>
<div>{children}</div>
</SectionContainer>
)
}
Configuring the Blog's Frontmatter
Use the layout
frontmatter field to specify the template to which the Markdown post should be mapped.
---
title: 如何使用该BLOG
date: 2024-07-01T15:32:14Z
lastmod: '2021-02-01'
tags: ['mdx','guide']
draft: false
summary: Nextjs + Tailwindcss + MDX 使用记录
layout: PostSimple
---
Traffic Analysis
Configure siteMetadata.js
with the settings corresponding to your preferred analytics provider.[TODO]
Comment System
The giscus commenting system is utilized, which can be configured on their website atgiscus. After configuring, the settings should be written into the siteMetadata.js
file.
Code Highlighting
The rehype-prism-plus plugin is used, which supports line highlighting and line numbering for code blocks.
python {1,3-4} showLineNumbers
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
If you wish to modify the styling, you can edit the prism.css
file.
.code-highlight {
@apply float-left min-w-full;
}
.code-line {
@apply -mx-4 block border-l-4 border-opacity-0 pl-4 pr-4;
}
.code-line.inserted {
@apply bg-green-500 bg-opacity-20;
}
.code-line.deleted {
@apply bg-red-500 bg-opacity-20;
}
.highlight-line {
@apply -mx-4 border-l-4 border-primary-500 bg-gray-700 bg-opacity-50;
}
.line-number::before {
@apply -ml-2 mr-4 inline-block w-4 text-right text-gray-400;
content: attr(line);
}
References system
Standard citation (Nash, 1950)
In-text citations e.g. Nash (1951)
Multiple citations (see Nash, 1950, 1951, p. 50)
References:
LaTeX Render
Mathematical expressions are parsed by remark-math
and rehype-katex
.1
Definition:
Assumptions:
- The definition above is linear.
- (conditional independence)
- rank() = (full rank)
- (homoscedasticity)
Objective:
Find a method to minimize the sum of squared errors for :
Solution:
Notice that is a scalar with symmetry .
Take the matrix derivative w.r.t :
Image Inclusion
Use Markdown syntax to include images, or directly utilize the Image component in Next.js.
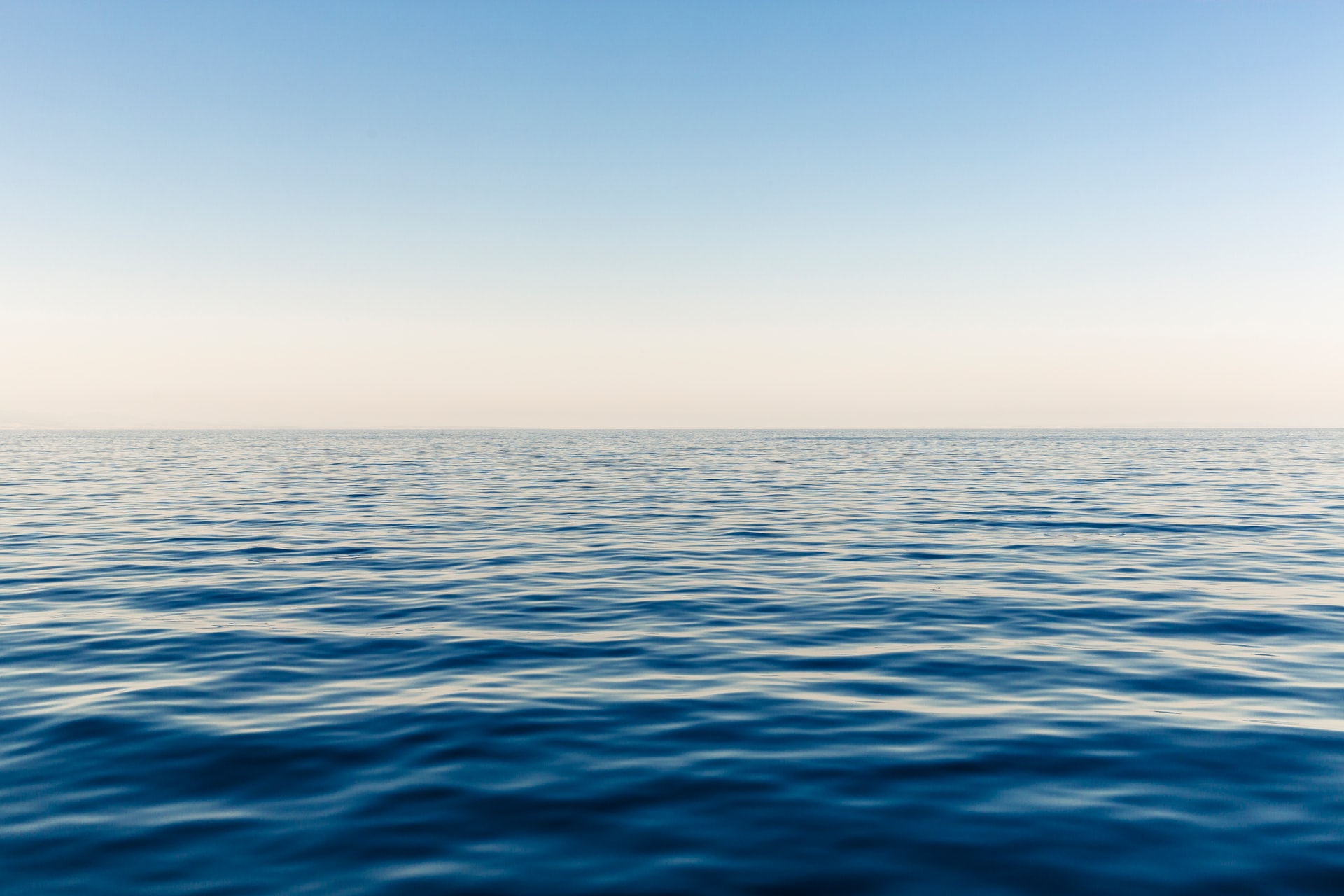
<Image alt="ocean" src="/static/images/ocean.jpg" width={256} height={128} />
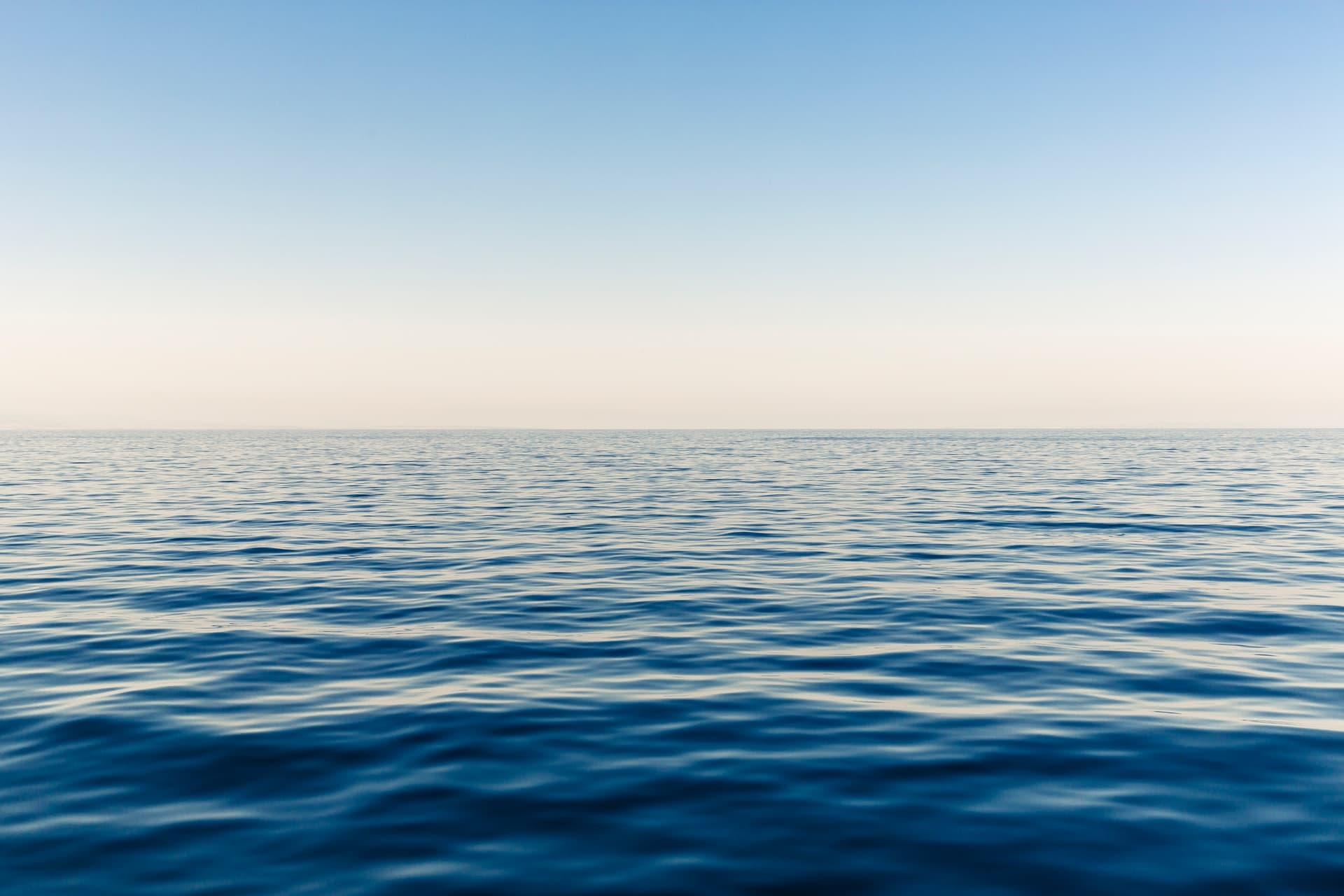
Footnotes
For a complete list of supported TeX functions, please refer to the [KaTeX documentation].(https://katex.org/docs/supported.html) ↩